Write your own Integer to String (itoa) converter.Implement your own Integer to ASCII (itoa) method which converts an Integer to String.Input 1: 123Output: "123" (as String)Input 2: 0Output: "0" (as String)Input 3: 8Output: "8" (as String)AlgorithmBefore...
Home
»
Archives for September 2020
Write your own String to Integer (atoi) implementation in Java
in
Interviews,
Miscellaneous
- on 00:07:00
- No comments
Write your own String to Integer (atoi) converter.Implement your own ASCII to Integer (atoi) method which converts a string to an integer.Input 1: "123"Output: 123 (as int)Input 2: "0"Output: 0 (as int)Input 3: "8"Output: 8 (as int)AlgorithmBefore we...
Merge all overlapping intervals in Java
in
Interviews
- on 17:22:00
- No comments

Merge overlapping intervals in Java.Given a N pairs of intervals. merge all overlapping intervals..You are given a pair of intervals in a format (start time, end time), Merge intervals that overlaps with each other.Consider this problem as asking someone...
Find overlapping interval among a given set of intervals.
in
Interviews
- on 22:44:00
- No comments
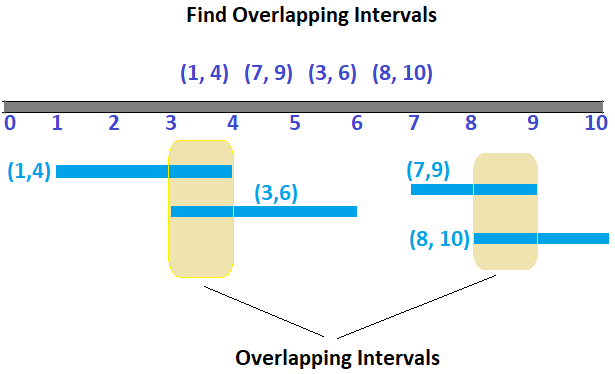
Find overlapping interval among a given set of intervals.Given a N pairs of intervals. Identify interval that overlap with other interval.You are given a pair of intervals in a format (start time, end time), find interval that overlaps with other interval.Example...
Serialize and Deserialize N-ary tree in Java
in
Datastructure,
Interviews,
N-ary-tree
- on 16:17:00
- No comments

Serialize and Deserialize N-ary tree in javaThis is a popular interview question asked in Tier-1 companies.Given an n-ary tree, serialize and deserialize it.Example of N-ary tree Serialization-Deserialization.Algorithm
Serialization and...
N-ary tree preorder traversal in java
in
Datastructure,
Interviews,
N-ary-tree
- on 14:52:00
- No comments

N-ary tree preorder traversal in javaThis is a popular interview question asked in Tier-1 companies.Given an n-ary tree, print preorder traversal of its nodes values.Example of N-ary tree preorder traversal below:Algorithm
Preorder traversal: To...
Minimum deletions required to make frequency of each letter unique in a String in Java
in
Array,
PriorityQueue
- on 20:10:00
- No comments
Minimum deletions to make frequency of each character uniqueThis is a popular interview question asked in Tier-1 companies.Given a string, find the minimum number of characters to be deleted in a string so that the frequency of each character in the...
Find longest binary gap in binary representation of integer number.
in
Interviews
- on 01:22:00
- No comments
Find longest length bi-valued slice in an array in java
This is a popular interview question asked in Tier-1 companies.
You are given a positive integer n, find the longest distance between two consecutive 1's in the binary representation of n.
Example:1....
Find longest length bi-valued slice in an array
in
Algorithm,
Array,
Interviews
- on 00:26:00
- No comments
Find longest length bi-valued slice in an array in java
This is a popular interview question asked in Tier-1 companies.
You are given a sequence of n integers and the task is to find the maximum slice of the array which contains no more than two...
Custom BlockingQueue implementation in java
in
Algorithm,
Datastructure,
Java
- on 23:27:00
- No comments

Producer Consumer using custom BlockingQueue implementation in java
Implement a custom Blocking Queue is very popular interview question.
What is Blocking Queue?
BlockingQueue is a queue data structure that blocks all the threads trying to...