How to construct a Binary Tree from given In order and Level order traversals.
Let us first understand what we want to achieve? what is the input and what will be the expected output?
Question:
Two traversals are given as input,
int[] inOrder...
Home
»
Archives for August 2015
Connect nodes at same level in a binary tree using constant extra space.
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 11:23:00
- No comments

How to connect nodes at same level using constant extra space? ORPopulate next right pointers for each Tree Node? ORConnect nodes of a binary tree at same Level?
Let us first understand what we want to achieve? what is the input and what will be the...
Connect nodes at same level in a Binary Tree.
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 14:15:00
- No comments

How to connect nodes at same level using Queue? ORPopulate next right pointers for each Tree Node? ORConnect nodes of a binary tree at same Level?
Let us first understand what we want to achieve? what is the input and what will be the expected output?
Question:...
Check if two trees are mirror images of each other
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure
- on 05:23:00
- 2 comments

How to check if two binary trees are mirror images of each other?
In this post we will look at algorithm to check if two trees are mirror images of each other with Java program.
See below image for better understanding of which Trees are called...
Check if two binary trees are identical
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure
- on 23:29:00
- 2 comments

Check if two binary trees are identical or not?
In this post we will check if given binary trees are identical or not using recursive approach.
See below image for better understanding of which Trees are called Identical and which not.
Check...
Binary Search Tree Operations ( Add a node in Binary Search Tree, Search a node in Binary Search Tree ).
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 02:18:00
- No comments
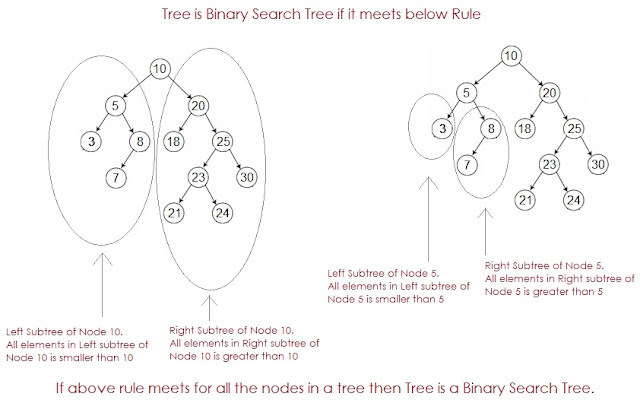
How to Add a Node and Search a Node in Binary Search Tree?
Let's see how to insert a node in binary search tree (BST) and search a node in binary search tree.
What is Binary Search Tree?
In computer science, Binary Search Trees (BST), sometimes...
Maximum Sum Contiguous Subarray using Kadane's algorithm.
in
Algorithm,
Array,
Datastructure,
Java
- on 03:44:00
- No comments
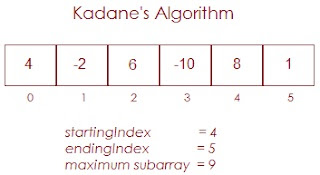
Find the maximum-sum subarray of an array. ORExplain Kadane's Algorithm to solve the Maximum Sum Subarray ORFind Largest Sum Contiguous Subarray.
The maximum subarray problem is the task of finding the contiguous subarray within a one-dimensional...
Delete a node in Binary Search Tree in Java
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 09:43:00
- No comments
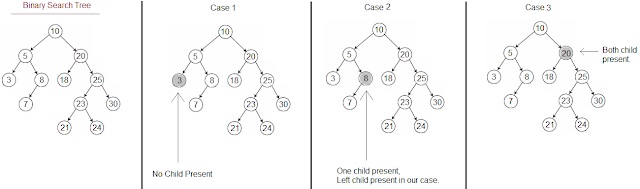
How to delete a Node in Binary Search Tree in Java?
Let's see how to delete a node in binary search tree with example and java program.
There are 3 cases that need to be considered while deleting a node from Binary Search Tree.
Node to delete...
Binary Tree Traversals - Inorder, Preorder, Postorder, Levelorder
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 14:25:00
- No comments
What is Binary Tree Traversal?
Binary tree traversal is a way to read a tree by visiting each node in a particular manner.
We will look into three popular binary tree traversal, Inorder, Preorder and Postorder along with example.
Note: Tree...
Add a node in Binary Tree and not a Binary Search Tree.
in
Algorithm,
Binary Search Tree,
Binary Tree,
Datastructure,
Java
- on 12:46:00
- No comments
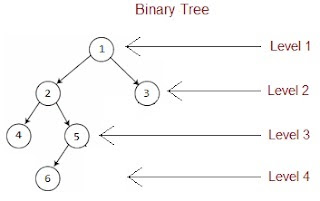
How to add a Node in Binary Tree and not a Binary Search Tree?
To add a Node in a Binary Tree, Let us first fix our protocol of how we are going a insert a node in Binary Tree.
Before going further, I would like to clear the difference between...
Print all possible strings of length K that can be formed from a set of N characters in Java
in
Algorithm,
Datastructure,
Java
- on 00:06:00
- No comments

Find all n length Permutation of a given String. OR Print all combinations/permutations of a string of length k that can be formed from a set of n characters. OR.Print all possible combinations of k elements from a given string of size n
Print all...
Write a program to print all permutations of a given string without repetition. (Repetition of characters is not allowed).
in
Algorithm,
Datastructure,
Java
- on 22:52:00
- 2 comments

Given a string of length n, print all permutation of the given string without Repetition . (Repetition of characters is NOT allowed)
Print all the permutations of a string without repetition in Java. lets understand with sample input and output below,
Case...